Note
Click here to download the full example code
Multi-Objective NAS with Ax
Created On: Aug 19, 2022 | Last Updated: Jul 31, 2024 | Last Verified: Nov 05, 2024
Authors: David Eriksson, Max Balandat, and the Adaptive Experimentation team at Meta.
In this tutorial, we show how to use Ax to run multi-objective neural architecture search (NAS) for a simple neural network model on the popular MNIST dataset. While the underlying methodology would typically be used for more complicated models and larger datasets, we opt for a tutorial that is easily runnable end-to-end on a laptop in less than 20 minutes.
In many NAS applications, there is a natural tradeoff between multiple objectives of interest. For instance, when deploying models on-device we may want to maximize model performance (for example, accuracy), while simultaneously minimizing competing metrics like power consumption, inference latency, or model size in order to satisfy deployment constraints. Often, we may be able to reduce computational requirements or latency of predictions substantially by accepting minimally lower model performance. Principled methods for exploring such tradeoffs efficiently are key enablers of scalable and sustainable AI, and have many successful applications at Meta - see for instance our case study on a Natural Language Understanding model.
In our example here, we will tune the widths of two hidden layers, the learning rate, the dropout probability, the batch size, and the number of training epochs. The goal is to trade off performance (accuracy on the validation set) and model size (the number of model parameters).
This tutorial makes use of the following PyTorch libraries:
PyTorch Lightning (specifying the model and training loop)
TorchX (for running training jobs remotely / asynchronously)
BoTorch (the Bayesian Optimization library powering Ax’s algorithms)
Defining the TorchX App
Our goal is to optimize the PyTorch Lightning training job defined in mnist_train_nas.py. To do this using TorchX, we write a helper function that takes in the values of the architecture and hyperparameters of the training job and creates a TorchX AppDef with the appropriate settings.
from pathlib import Path
import torchx
from torchx import specs
from torchx.components import utils
def trainer(
log_path: str,
hidden_size_1: int,
hidden_size_2: int,
learning_rate: float,
epochs: int,
dropout: float,
batch_size: int,
trial_idx: int = -1,
) -> specs.AppDef:
# define the log path so we can pass it to the TorchX ``AppDef``
if trial_idx >= 0:
log_path = Path(log_path).joinpath(str(trial_idx)).absolute().as_posix()
return utils.python(
# command line arguments to the training script
"--log_path",
log_path,
"--hidden_size_1",
str(hidden_size_1),
"--hidden_size_2",
str(hidden_size_2),
"--learning_rate",
str(learning_rate),
"--epochs",
str(epochs),
"--dropout",
str(dropout),
"--batch_size",
str(batch_size),
# other config options
name="trainer",
script="mnist_train_nas.py",
image=torchx.version.TORCHX_IMAGE,
)
Setting up the Runner
Ax’s Runner abstraction allows writing interfaces to various backends. Ax already comes with Runner for TorchX, and so we just need to configure it. For the purpose of this tutorial we run jobs locally in a fully asynchronous fashion.
In order to launch them on a cluster, you can instead specify a
different TorchX scheduler and adjust the configuration appropriately.
For example, if you have a Kubernetes cluster, you just need to change the
scheduler from local_cwd
to kubernetes
).
import tempfile
from ax.runners.torchx import TorchXRunner
# Make a temporary dir to log our results into
log_dir = tempfile.mkdtemp()
ax_runner = TorchXRunner(
tracker_base="/tmp/",
component=trainer,
# NOTE: To launch this job on a cluster instead of locally you can
# specify a different scheduler and adjust arguments appropriately.
scheduler="local_cwd",
component_const_params={"log_path": log_dir},
cfg={},
)
Setting up the SearchSpace
First, we define our search space. Ax supports both range parameters of type integer and float as well as choice parameters which can have non-numerical types such as strings. We will tune the hidden sizes, learning rate, dropout, and number of epochs as range parameters and tune the batch size as an ordered choice parameter to enforce it to be a power of 2.
from ax.core import (
ChoiceParameter,
ParameterType,
RangeParameter,
SearchSpace,
)
parameters = [
# NOTE: In a real-world setting, hidden_size_1 and hidden_size_2
# should probably be powers of 2, but in our simple example this
# would mean that ``num_params`` can't take on that many values, which
# in turn makes the Pareto frontier look pretty weird.
RangeParameter(
name="hidden_size_1",
lower=16,
upper=128,
parameter_type=ParameterType.INT,
log_scale=True,
),
RangeParameter(
name="hidden_size_2",
lower=16,
upper=128,
parameter_type=ParameterType.INT,
log_scale=True,
),
RangeParameter(
name="learning_rate",
lower=1e-4,
upper=1e-2,
parameter_type=ParameterType.FLOAT,
log_scale=True,
),
RangeParameter(
name="epochs",
lower=1,
upper=4,
parameter_type=ParameterType.INT,
),
RangeParameter(
name="dropout",
lower=0.0,
upper=0.5,
parameter_type=ParameterType.FLOAT,
),
ChoiceParameter( # NOTE: ``ChoiceParameters`` don't require log-scale
name="batch_size",
values=[32, 64, 128, 256],
parameter_type=ParameterType.INT,
is_ordered=True,
sort_values=True,
),
]
search_space = SearchSpace(
parameters=parameters,
# NOTE: In practice, it may make sense to add a constraint
# hidden_size_2 <= hidden_size_1
parameter_constraints=[],
)
Setting up Metrics
Ax has the concept of a Metric that defines properties of outcomes and how observations are obtained for these outcomes. This allows e.g. encoding how data is fetched from some distributed execution backend and post-processed before being passed as input to Ax.
In this tutorial we will use multi-objective optimization with the goal of maximizing the validation accuracy and minimizing the number of model parameters. The latter represents a simple proxy of model latency, which is hard to estimate accurately for small ML models (in an actual application we would benchmark the latency while running the model on-device).
In our example TorchX will run the training jobs in a fully asynchronous
fashion locally and write the results to the log_dir
based on the trial
index (see the trainer()
function above). We will define a metric
class that is aware of that logging directory. By subclassing
TensorboardCurveMetric
we get the logic to read and parse the TensorBoard logs for free.
from ax.metrics.tensorboard import TensorboardMetric
from tensorboard.backend.event_processing import plugin_event_multiplexer as event_multiplexer
class MyTensorboardMetric(TensorboardMetric):
# NOTE: We need to tell the new TensorBoard metric how to get the id /
# file handle for the TensorBoard logs from a trial. In this case
# our convention is to just save a separate file per trial in
# the prespecified log dir.
def _get_event_multiplexer_for_trial(self, trial):
mul = event_multiplexer.EventMultiplexer(max_reload_threads=20)
mul.AddRunsFromDirectory(Path(log_dir).joinpath(str(trial.index)).as_posix(), None)
mul.Reload()
return mul
# This indicates whether the metric is queryable while the trial is
# still running. We don't use this in the current tutorial, but Ax
# utilizes this to implement trial-level early-stopping functionality.
@classmethod
def is_available_while_running(cls):
return False
Now we can instantiate the metrics for accuracy and the number of model parameters. Here curve_name is the name of the metric in the TensorBoard logs, while name is the metric name used internally by Ax. We also specify lower_is_better to indicate the favorable direction of the two metrics.
val_acc = MyTensorboardMetric(
name="val_acc",
tag="val_acc",
lower_is_better=False,
)
model_num_params = MyTensorboardMetric(
name="num_params",
tag="num_params",
lower_is_better=True,
)
Setting up the OptimizationConfig
The way to tell Ax what it should optimize is by means of an
OptimizationConfig.
Here we use a MultiObjectiveOptimizationConfig
as we will
be performing multi-objective optimization.
Additionally, Ax supports placing constraints on the different metrics by specifying objective thresholds, which bound the region of interest in the outcome space that we want to explore. For this example, we will constrain the validation accuracy to be at least 0.94 (94%) and the number of model parameters to be at most 80,000.
from ax.core import MultiObjective, Objective, ObjectiveThreshold
from ax.core.optimization_config import MultiObjectiveOptimizationConfig
opt_config = MultiObjectiveOptimizationConfig(
objective=MultiObjective(
objectives=[
Objective(metric=val_acc, minimize=False),
Objective(metric=model_num_params, minimize=True),
],
),
objective_thresholds=[
ObjectiveThreshold(metric=val_acc, bound=0.94, relative=False),
ObjectiveThreshold(metric=model_num_params, bound=80_000, relative=False),
],
)
Creating the Ax Experiment
In Ax, the Experiment object is the object that stores all the information about the problem setup.
from ax.core import Experiment
experiment = Experiment(
name="torchx_mnist",
search_space=search_space,
optimization_config=opt_config,
runner=ax_runner,
)
Choosing the Generation Strategy
A GenerationStrategy is the abstract representation of how we would like to perform the optimization. While this can be customized (if you’d like to do so, see this tutorial), in most cases Ax can automatically determine an appropriate strategy based on the search space, optimization config, and the total number of trials we want to run.
Typically, Ax chooses to evaluate a number of random configurations before starting a model-based Bayesian Optimization strategy.
total_trials = 48 # total evaluation budget
from ax.modelbridge.dispatch_utils import choose_generation_strategy
gs = choose_generation_strategy(
search_space=experiment.search_space,
optimization_config=experiment.optimization_config,
num_trials=total_trials,
)
[INFO 04-09 21:48:51] ax.modelbridge.dispatch_utils: Using Models.BOTORCH_MODULAR since there is at least one ordered parameter and there are no unordered categorical parameters.
[INFO 04-09 21:48:51] ax.modelbridge.dispatch_utils: Calculating the number of remaining initialization trials based on num_initialization_trials=None max_initialization_trials=None num_tunable_parameters=6 num_trials=48 use_batch_trials=False
[INFO 04-09 21:48:51] ax.modelbridge.dispatch_utils: calculated num_initialization_trials=9
[INFO 04-09 21:48:51] ax.modelbridge.dispatch_utils: num_completed_initialization_trials=0 num_remaining_initialization_trials=9
[INFO 04-09 21:48:51] ax.modelbridge.dispatch_utils: `verbose`, `disable_progbar`, and `jit_compile` are not yet supported when using `choose_generation_strategy` with ModularBoTorchModel, dropping these arguments.
[INFO 04-09 21:48:51] ax.modelbridge.dispatch_utils: Using Bayesian Optimization generation strategy: GenerationStrategy(name='Sobol+BoTorch', steps=[Sobol for 9 trials, BoTorch for subsequent trials]). Iterations after 9 will take longer to generate due to model-fitting.
Configuring the Scheduler
The Scheduler
acts as the loop control for the optimization.
It communicates with the backend to launch trials, check their status,
and retrieve results. In the case of this tutorial, it is simply reading
and parsing the locally saved logs. In a remote execution setting,
it would call APIs. The following illustration from the Ax
Scheduler tutorial
summarizes how the Scheduler interacts with external systems used to run
trial evaluations:
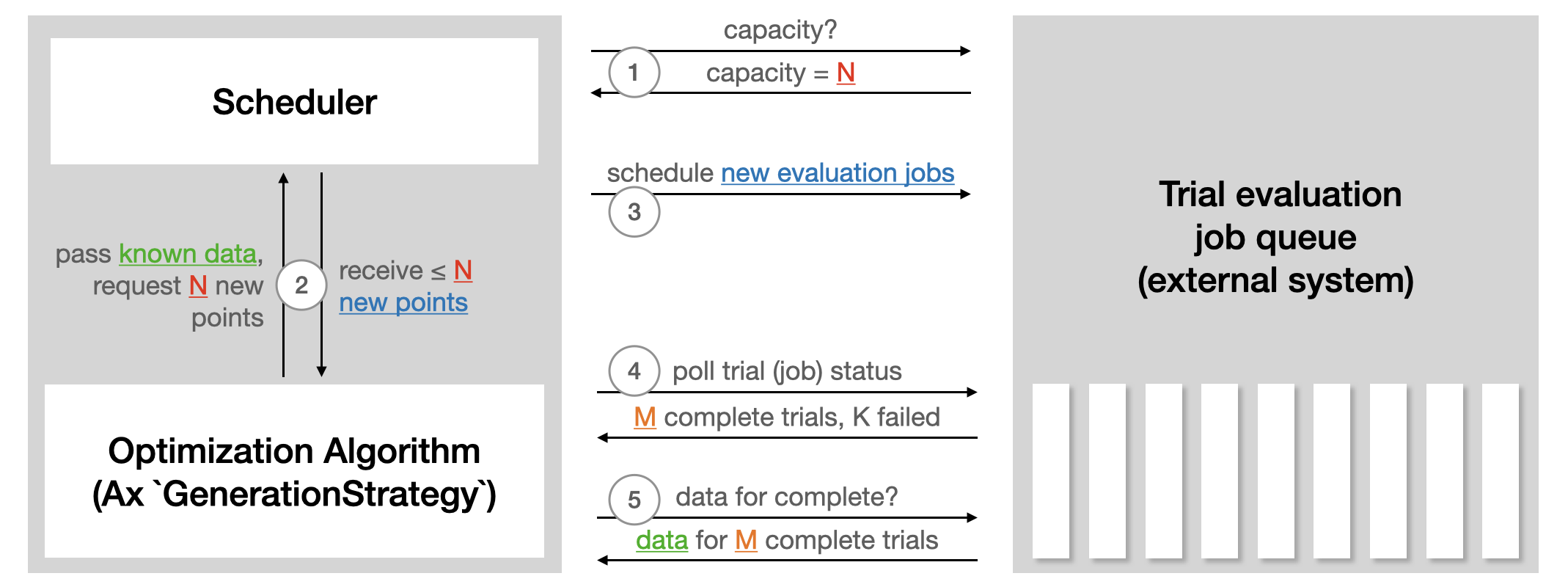
The Scheduler
requires the Experiment
and the GenerationStrategy
.
A set of options can be passed in via SchedulerOptions
. Here, we
configure the number of total evaluations as well as max_pending_trials
,
the maximum number of trials that should run concurrently. In our
local setting, this is the number of training jobs running as individual
processes, while in a remote execution setting, this would be the number
of machines you want to use in parallel.
from ax.service.scheduler import Scheduler, SchedulerOptions
scheduler = Scheduler(
experiment=experiment,
generation_strategy=gs,
options=SchedulerOptions(
total_trials=total_trials, max_pending_trials=4
),
)
[WARNING 04-09 21:48:51] ax.service.utils.with_db_settings_base: Ax currently requires a sqlalchemy version below 2.0. This will be addressed in a future release. Disabling SQL storage in Ax for now, if you would like to use SQL storage please install Ax with mysql extras via `pip install ax-platform[mysql]`.
[INFO 04-09 21:48:51] Scheduler: `Scheduler` requires experiment to have immutable search space and optimization config. Setting property immutable_search_space_and_opt_config to `True` on experiment.
Running the optimization
Now that everything is configured, we can let Ax run the optimization in a fully automated fashion. The Scheduler will periodically check the logs for the status of all currently running trials, and if a trial completes the scheduler will update its status on the experiment and fetch the observations needed for the Bayesian optimization algorithm.
scheduler.run_all_trials()
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:48:51] Scheduler: Running trials [0]...
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:48:51] Scheduler: Running trials [1]...
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:48:52] Scheduler: Running trials [2]...
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:48:53] Scheduler: Running trials [3]...
[INFO 04-09 21:48:54] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:48:55] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:48:57] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 4).
[INFO 04-09 21:48:59] Scheduler: Retrieved FAILED trials: [0].
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:48:59] Scheduler: Running trials [4]...
[INFO 04-09 21:49:00] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:49:01] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:49:03] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 4).
[INFO 04-09 21:49:05] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 4).
[INFO 04-09 21:49:08] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 4).
[INFO 04-09 21:49:13] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 4).
[INFO 04-09 21:49:21] Scheduler: Retrieved COMPLETED trials: [3].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:49:21] Scheduler: Running trials [5]...
[INFO 04-09 21:49:22] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:49:23] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:49:25] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 4).
[INFO 04-09 21:49:27] Scheduler: Retrieved COMPLETED trials: [1].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:49:27] Scheduler: Running trials [6]...
[INFO 04-09 21:49:27] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:49:28] Scheduler: Retrieved COMPLETED trials: [4].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:49:28] Scheduler: Running trials [7]...
[INFO 04-09 21:49:29] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:49:30] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:49:32] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 4).
[INFO 04-09 21:49:34] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 4).
[INFO 04-09 21:49:37] Scheduler: Retrieved COMPLETED trials: [2].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:49:37] Scheduler: Running trials [8]...
[INFO 04-09 21:49:38] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:49:39] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:49:41] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 4).
[INFO 04-09 21:49:43] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 4).
[INFO 04-09 21:49:46] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 4).
[INFO 04-09 21:49:51] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 4).
[INFO 04-09 21:49:59] Scheduler: Retrieved COMPLETED trials: [6].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/modelbridge/cross_validation.py:439: UserWarning:
Encountered exception in computing model fit quality: RandomModelBridge does not support prediction.
[INFO 04-09 21:49:59] Scheduler: Running trials [9]...
[INFO 04-09 21:50:00] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:50:01] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:50:03] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 4).
[INFO 04-09 21:50:05] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 4).
[INFO 04-09 21:50:08] Scheduler: Retrieved COMPLETED trials: [5].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:50:17] Scheduler: Running trials [10]...
[INFO 04-09 21:50:18] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:50:19] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 4).
[INFO 04-09 21:50:20] Scheduler: Retrieved COMPLETED trials: [9].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:50:30] Scheduler: Running trials [11]...
[INFO 04-09 21:50:30] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 4).
[INFO 04-09 21:50:31] Scheduler: Retrieved COMPLETED trials: [8].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:50:38] Scheduler: Running trials [12]...
[INFO 04-09 21:50:38] Scheduler: Retrieved COMPLETED trials: [7].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:50:40] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:50:41] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:50:42] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:50:45] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:50:48] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:50:53] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:51:01] Scheduler: Retrieved COMPLETED trials: [10].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:51:11] Scheduler: Running trials [13]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:51:12] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:51:12] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:51:13] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:51:14] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:51:17] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:51:20] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:51:25] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:51:33] Scheduler: Retrieved COMPLETED trials: [12].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:51:43] Scheduler: Running trials [14]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:51:44] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:51:44] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:51:45] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:51:46] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:51:49] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:51:52] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:51:57] Scheduler: Retrieved COMPLETED trials: [11].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:52:06] Scheduler: Running trials [15]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:52:07] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:52:07] Scheduler: Retrieved COMPLETED trials: [13].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:52:18] Scheduler: Running trials [16]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:52:19] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:52:19] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:52:20] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:52:21] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:52:23] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:52:27] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:52:32] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:52:39] Scheduler: Retrieved COMPLETED trials: [14].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:52:51] Scheduler: Running trials [17]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:52:51] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:52:51] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:52:52] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:52:54] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:52:56] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:52:59] Scheduler: Retrieved COMPLETED trials: [16].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:53:11] Scheduler: Running trials [18]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:53:12] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:53:12] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:53:13] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:53:15] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:53:17] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:53:20] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:53:25] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:53:33] Scheduler: Retrieved COMPLETED trials: [15].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:53:44] Scheduler: Running trials [19]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:53:45] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:53:45] Scheduler: Retrieved COMPLETED trials: [17].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:53:57] Scheduler: Running trials [20]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:53:58] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:53:58] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:53:59] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:54:01] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:54:03] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:54:06] Scheduler: Retrieved COMPLETED trials: [18].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:54:19] Scheduler: Running trials [21]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:54:19] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:54:19] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:54:20] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:54:22] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:54:24] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:54:27] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:54:32] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:54:40] Scheduler: Retrieved COMPLETED trials: [19].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:54:52] Scheduler: Running trials [22]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:54:54] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:54:54] Scheduler: Retrieved COMPLETED trials: [20].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:55:09] Scheduler: Running trials [23]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:55:09] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:55:09] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:55:10] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:55:12] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:55:14] Scheduler: Retrieved COMPLETED trials: [21].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:55:30] Scheduler: Running trials [24]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:55:31] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:55:31] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:55:32] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:55:34] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:55:36] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:55:39] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:55:44] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:55:52] Scheduler: Retrieved COMPLETED trials: [22].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:56:04] Scheduler: Running trials [25]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:56:05] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:56:05] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:56:06] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:56:08] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:56:10] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:56:13] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:56:19] Scheduler: Retrieved COMPLETED trials: [24].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:56:35] Scheduler: Running trials [26]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:56:36] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:56:36] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:56:37] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:56:39] Scheduler: Retrieved COMPLETED trials: [23].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:56:56] Scheduler: Running trials [27]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:56:57] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:56:57] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:56:58] Scheduler: Retrieved COMPLETED trials: [25].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:57:11] Scheduler: Running trials [28]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:57:12] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:57:12] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:57:13] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:57:14] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:57:17] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:57:20] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:57:25] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:57:33] Scheduler: Retrieved COMPLETED trials: [26].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:57:47] Scheduler: Running trials [29]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:57:48] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:57:48] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:57:49] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:57:51] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:57:53] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:57:57] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:58:02] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:58:09] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 3).
[INFO 04-09 21:58:21] Scheduler: Retrieved COMPLETED trials: [27].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:58:34] Scheduler: Running trials [30]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:58:35] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:58:35] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:58:36] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:58:38] Scheduler: Retrieved COMPLETED trials: [28].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:58:52] Scheduler: Running trials [31]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:58:53] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:58:53] Scheduler: Retrieved COMPLETED trials: [29].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:59:06] Scheduler: Running trials [32]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:59:07] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:59:07] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 21:59:08] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 21:59:10] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 21:59:12] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 21:59:16] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 21:59:21] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 21:59:28] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 3).
[INFO 04-09 21:59:40] Scheduler: Retrieved COMPLETED trials: [30].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 21:59:52] Scheduler: Running trials [33]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 21:59:52] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 21:59:52] Scheduler: Retrieved COMPLETED trials: [31].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:00:13] Scheduler: Running trials [34]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:00:13] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:00:13] Scheduler: Retrieved COMPLETED trials: [32].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:00:28] Scheduler: Running trials [35]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:00:29] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:00:29] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 22:00:30] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 22:00:32] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 22:00:34] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 22:00:37] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 22:00:42] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 22:00:50] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 3).
[INFO 04-09 22:01:02] Scheduler: Retrieved COMPLETED trials: [33].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:01:19] Scheduler: Running trials [36]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:01:20] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:01:20] Scheduler: Retrieved COMPLETED trials: [34].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:01:36] Scheduler: Running trials [37]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:01:37] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:01:37] Scheduler: Retrieved COMPLETED trials: [35].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:01:55] Scheduler: Running trials [38]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:01:56] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:01:56] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 22:01:57] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 22:01:58] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 22:02:00] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 22:02:04] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 22:02:09] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 22:02:17] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 3).
[INFO 04-09 22:02:28] Scheduler: Retrieved COMPLETED trials: [36].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:02:44] Scheduler: Running trials [39]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:02:45] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:02:45] Scheduler: Retrieved COMPLETED trials: 37 - 38.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:03:07] Scheduler: Running trials [40]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:03:15] Scheduler: Running trials [41]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:03:17] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:03:17] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 22:03:18] Scheduler: Retrieved COMPLETED trials: [39].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:03:44] Scheduler: Running trials [42]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:03:45] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:03:45] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 22:03:46] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 22:03:47] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 22:03:49] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 22:03:53] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 22:03:58] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 22:04:05] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 3).
[INFO 04-09 22:04:17] Scheduler: Waiting for completed trials (for 17 sec, currently running trials: 3).
[INFO 04-09 22:04:34] Scheduler: Retrieved COMPLETED trials: [40].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:04:56] Scheduler: Running trials [43]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:04:57] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:04:57] Scheduler: Retrieved COMPLETED trials: 41 - 42.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:05:17] Scheduler: Running trials [44]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:05:23] Scheduler: Running trials [45]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:05:24] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:05:24] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 3).
[INFO 04-09 22:05:25] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 3).
[INFO 04-09 22:05:27] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 3).
[INFO 04-09 22:05:29] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 3).
[INFO 04-09 22:05:33] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 3).
[INFO 04-09 22:05:38] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 3).
[INFO 04-09 22:05:45] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 3).
[INFO 04-09 22:05:57] Scheduler: Retrieved COMPLETED trials: [43].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:06:16] Scheduler: Running trials [46]...
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:06:17] Scheduler: Generated all trials that can be generated currently. Max parallelism currently reached.
[INFO 04-09 22:06:17] Scheduler: Retrieved COMPLETED trials: [44].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
/usr/local/lib/python3.10/dist-packages/botorch/optim/optimize_mixed.py:702: OptimizationWarning:
Failed to initialize using continuous relaxation. Using `sample_feasible_points` for initialization. Original error message: split() missing required argument 'split_size_or_sections' (pos 2)
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
/usr/lib/python3.10/dataclasses.py:1453: RuntimeWarning:
If using a 2-dim `batch_initial_conditions` botorch will default to old behavior of ignoring `num_restarts` and just use the given `batch_initial_conditions` by setting `raw_samples` to None.
[INFO 04-09 22:06:35] Scheduler: Running trials [47]...
[INFO 04-09 22:06:36] Scheduler: Retrieved COMPLETED trials: [45].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:06:36] Scheduler: Done submitting trials, waiting for remaining 2 running trials...
[INFO 04-09 22:06:36] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 2).
[INFO 04-09 22:06:37] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 2).
[INFO 04-09 22:06:39] Scheduler: Waiting for completed trials (for 2 sec, currently running trials: 2).
[INFO 04-09 22:06:41] Scheduler: Waiting for completed trials (for 3 sec, currently running trials: 2).
[INFO 04-09 22:06:44] Scheduler: Waiting for completed trials (for 5 sec, currently running trials: 2).
[INFO 04-09 22:06:49] Scheduler: Waiting for completed trials (for 7 sec, currently running trials: 2).
[INFO 04-09 22:06:57] Scheduler: Waiting for completed trials (for 11 sec, currently running trials: 2).
[INFO 04-09 22:07:08] Scheduler: Waiting for completed trials (for 17 sec, currently running trials: 2).
[INFO 04-09 22:07:25] Scheduler: Retrieved COMPLETED trials: [46].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
[INFO 04-09 22:07:25] Scheduler: Waiting for completed trials (for 1 sec, currently running trials: 1).
[INFO 04-09 22:07:26] Scheduler: Waiting for completed trials (for 1.5 sec, currently running trials: 1).
[INFO 04-09 22:07:28] Scheduler: Retrieved COMPLETED trials: [47].
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
OptimizationResult()
Evaluating the results
We can now inspect the result of the optimization using helper functions and visualizations included with Ax.
First, we generate a dataframe with a summary of the results of the experiment. Each row in this dataframe corresponds to a trial (that is, a training job that was run), and contains information on the status of the trial, the parameter configuration that was evaluated, and the metric values that were observed. This provides an easy way to sanity check the optimization.
from ax.service.utils.report_utils import exp_to_df
df = exp_to_df(experiment)
df.head(10)
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
We can also visualize the Pareto frontier of tradeoffs between the validation accuracy and the number of model parameters.
Tip
Ax uses Plotly to produce interactive plots, which allow you to do things like zoom, crop, or hover in order to view details of components of the plot. Try it out, and take a look at the visualization tutorial if you’d like to learn more).
The final optimization results are shown in the figure below where the color corresponds to the iteration number for each trial. We see that our method was able to successfully explore the trade-offs and found both large models with high validation accuracy as well as small models with comparatively lower validation accuracy.
from ax.service.utils.report_utils import _pareto_frontier_scatter_2d_plotly
_pareto_frontier_scatter_2d_plotly(experiment)
/usr/local/lib/python3.10/dist-packages/ax/core/map_data.py:216: FutureWarning:
The behavior of DataFrame concatenation with empty or all-NA entries is deprecated. In a future version, this will no longer exclude empty or all-NA columns when determining the result dtypes. To retain the old behavior, exclude the relevant entries before the concat operation.
To better understand what our surrogate models have learned about the black box objectives, we can take a look at the leave-one-out cross validation results. Since our models are Gaussian Processes, they not only provide point predictions but also uncertainty estimates about these predictions. A good model means that the predicted means (the points in the figure) are close to the 45 degree line and that the confidence intervals cover the 45 degree line with the expected frequency (here we use 95% confidence intervals, so we would expect them to contain the true observation 95% of the time).
As the figures below show, the model size (num_params
) metric is
much easier to model than the validation accuracy (val_acc
) metric.
from ax.modelbridge.cross_validation import compute_diagnostics, cross_validate
from ax.plot.diagnostic import interact_cross_validation_plotly
from ax.utils.notebook.plotting import init_notebook_plotting, render
cv = cross_validate(model=gs.model) # The surrogate model is stored on the ``GenerationStrategy``
compute_diagnostics(cv)
interact_cross_validation_plotly(cv)
We can also make contour plots to better understand how the different objectives depend on two of the input parameters. In the figure below, we show the validation accuracy predicted by the model as a function of the two hidden sizes. The validation accuracy clearly increases as the hidden sizes increase.
from ax.plot.contour import interact_contour_plotly
interact_contour_plotly(model=gs.model, metric_name="val_acc")
Similarly, we show the number of model parameters as a function of
the hidden sizes in the figure below and see that it also increases
as a function of the hidden sizes (the dependency on hidden_size_1
is much larger).
interact_contour_plotly(model=gs.model, metric_name="num_params")